I’ve not fully explored all there is in the Full Site Editing (FSE) experience that WordPress has released over the past two or three versions. But one thing that I noticed right away was the default footer template was the Copyright date was just manually editable text, and not automatically updated each year.
I felt a footer that automatically updated the Copyright date was desperately needed. So I built the Copyright Footer plugin.
If you want to dig into the code, you can find it on Github.
In this post, I’ll talk a little bit about the approach to coding this as a standalone block plugin.
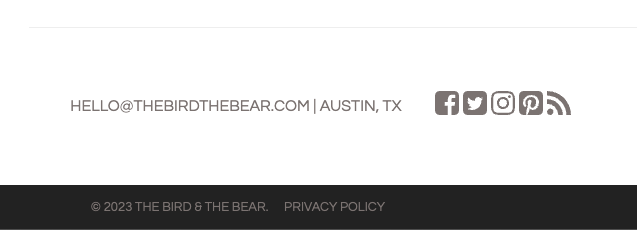
FSE is of course block based, and with native blocks being built in Javascript and output to content, it makes sense why they didn’t think to build the default theme with something custom to update the date.
But as a long time custom WordPress theme developer, I wanted to find out a way to update the Copyright Year like I do with all my sites. This was going to be a pretty simple plugin, and I thought it would have a lot of appeal, so I made the decision to make it available for free in the WordPress Plugin Repo. And since individual blocks can now be uploaded to the Plugin Repo, it made even more sense.
The Plugin
The initial objective was to make a footer that automatically updated the date shown. This couldn’t be done through via React in the Save.js or Edit.js as there’s a build process and the JS doesn’t actually run anything on the frontend.
So instead, I used a filter to run a PHP str_replace()
on the date. You can see the filter here in the main plugin file:
function copyright_footer_replace_year_filter( $block_content, $block ) {
$year = date( 'Y' );
$site_name = get_bloginfo( 'name' );
if ( $block['blockName'] !== 'fys/copyright-footer') {
return $block_content;
}
$block_content = str_replace( "%SITENAME%", esc_html( $site_name ), $block_content );
$block_content = str_replace( "%YEAR%", $year, $block_content );
return $block_content;
}
add_filter( 'render_block', 'copyright_footer_replace_year_filter', 10, 2 );
The function runs on the render_block()
hook, and on line 2 it uses the PHP date()
function to define the current year date. (On line 3 it checks the Site Name in the event it has been changed).
On line 5 I run a check to ensure it’s the Copyright Footer block (if it’s not it returns the content without any filtering).
On lines 9 & 10 we run the str_replace()
on the predefined text that has been coded in the Save.js file %SITENAME%
and %YEAR%.
You can see below on line 4.
return (
<div {...blockProps}>
<p className="copyright">
© {toggleYear && "%YEAR%"} {toggleSite && "%SITENAME%"}
{toggleLegalLinks && " | "}
{toggleLegalLinks && (
<RichText.Content tagName="span" value={legalLinks} />
)}
</p>
{toggleSiteCredit && <RichText.Content tagName="p" value={siteCredit} />}
</div>
);
Dynamically loading data into Edit.js
For the Edit.js, we want to display the Date and Site Name dynamically with Javascript in the Block Editor. So we need to run a couple JS functions to get those.
The first (Line 1 below), we just use a native Date() method to get the full year. Easy Peasy.
The second was a bit harder to figure out. At first I thought about loading the REST API, but after digging a bit, I found the WordPress Data Module. When it’s imported into your React files, you can access certain endpoints directly. (Here’s more info about the WordPress Data Module).
You can see on line 2 below that I’m selecting the Core namespace, and digging down to the Site Title.
const year = new Date().getFullYear();
const siteName = wp.data.select("core").getSite().title;
Further down in the Edit.js file we add those variables into the output (line 3).
<div {...blockProps}>
<p className="copyright">
© {toggleYear && year} {toggleSite && siteName}
{toggleLegalLinks && " | "}
{toggleLegalLinks && (
<RichText
tagName="span"
className="legal-link"
value={legalLinks}
onChange={(legalLinks) => setAttributes({ legalLinks })}
placeholder="Click here to edit. (Optional)"
/>
)}
</p>
Result
So, there it is. On the front end of the site, the Date and the Site Name are filtered using PHP functions.
And while editing the Footer in the block editor where React is being loaded and we can run JS functions, we’re using Javascript to dynamically pull the in formation.
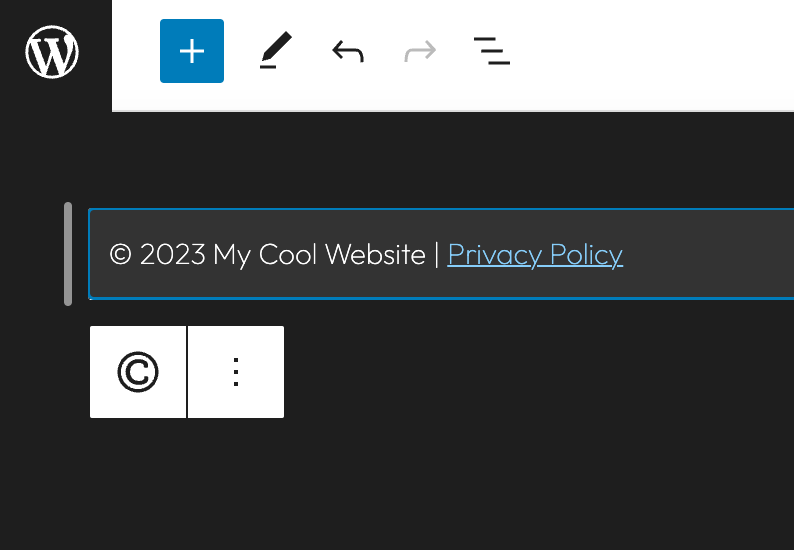
Leave a Reply